Xamarin.Forms - Network Speed Monitor
Xamarin.Forms - Network Speed Monitor
Introduction
Xamarin.Forms code runs on multiple platforms - each of which has its own filesystem. This means that reading and writing files is most easily done using the native file APIs on each platform. Alternatively, embedded resources are a simpler solution to distribute data files with an app.
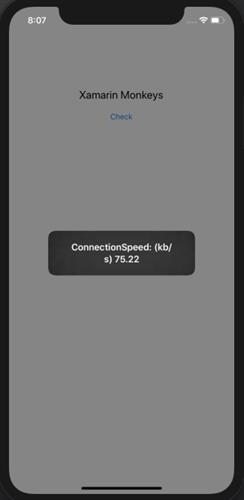
Prerequisites
- Visual Studio 2017 or later (Windows or Mac)
Setting up a Xamarin.Forms Project
Start by creating a new Xamarin.Forms project. You wíll learn more by going through the steps yourself.
Create a new or existing Xamarin forms(.Net standard) Project. With Android and iOS Platform.
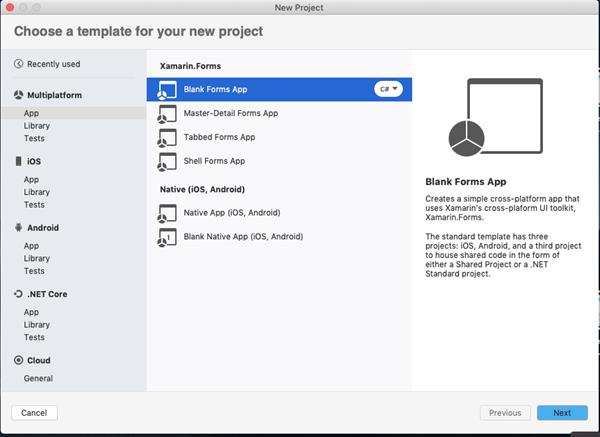
Network Monitor
Now, create a network that helps the class to check network connectivity in xamarin.froms.
NetworkHelper.cs
public class NetworkHelper
{
public async Task<string> CheckInternetSpeed()
{
DateTime dt1 = DateTime.Now;
string internetSpeed;
try
{
var client = new HttpClient();
byte[] data = await client.GetByteArrayAsync("http://xamarinmonkeys.blogspot.com/");
DateTime dt2 = DateTime.Now; Console.WriteLine("ConnectionSpeed: DataSize (kb) " + data.Length / 1024);
Console.WriteLine("ConnectionSpeed: ElapsedTime (secs) " + (dt2 - dt1).TotalSeconds);
internetSpeed = "ConnectionSpeed: (kb/s) " + Math.Round((data.Length / 1024) / (dt2 - dt1).TotalSeconds, 2);
}
catch (Exception ex)
{
internetSpeed = "ConnectionSpeed:Unknown Exception-" + ex.Message;
}
Console.WriteLine(internetSpeed);
return internetSpeed;
}
}
Consuming Network helper
Here, you will call the Network helper class and you will get the network speed.
MainPage.Xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="NetworkPOC.MainPage">
<StackLayout HorizontalOptions="Center" VerticalOptions="Start" Margin="0,150,0,0">
<Label FontSize="Large" Text="Xamarin Monkeys"/>
<Button Text="Check" Clicked="Button_Clicked"></Button>
</StackLayout>
</ContentPage>
Here I show the result in a toast.
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Xamarin.Forms;
using Xamarin.Essentials;
using System.Net.Http;
namespace NetworkPOC
{
// Learn more about making custom code visible in the Xamarin.Forms previewer
// by visiting https://aka.ms/xamarinforms-previewer
[DesignTimeVisible(false)]
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private async void Button_Clicked(object sender, EventArgs e)
{
if (current == NetworkAccess.Internet)
{
var speed=await CheckInternetSpeed();
DependencyService.Get<IToast>().ShowToast(speed);
}
else
{
DependencyService.Get<IToast>().ShowToast("No Internet Connection");
}
}
}
}
Run
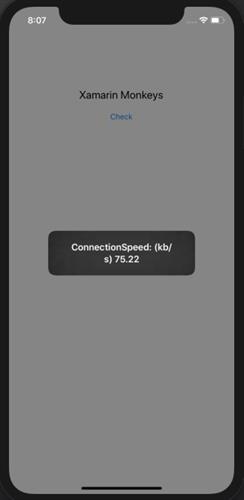
I hope you have understood you will learn how to check network speed in Xamarin.Forms.
Thanks for reading. Please share your comments and feedback.
Happy Coding :)