- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
do while Repetition Statement
The do…while repetition statement is similar to the while statement. In the while statement, the loop-continuation condition is tested at the beginning of the loop before the body of the loop is performed. The do…while statement tests the loop-continuation condition after the loop body is performed. Therefore, the loop body will be executed at least
once. When a do…while terminates, execution continues with the statement after the while clause. It’s not necessary to use braces in the do…while statement if there is only one statement in the body. However, the braces are usually included to avoid confusion between the while and do…while statements. For example,
while ( condition )
is normally regarded as the header to a while statement. A do…while with no braces around the single-statement body appears as
do
statement
while ( condition );
which can be confusing. The last line while( condition ); may be misinterpreted by as a while statement containing an empty statement. Thus, to avoid confusion, the do…while with one statement is often written as follows:
do {
statement
} while ( condition );
To eliminate the potential for ambiguity, some programmers always include braces in a do…while statement, even if the braces are not necessary. Infinite loops are caused when the loop-continuation condition in a while, for or do…while statement never becomes false. To prevent this, make sure there is not a semicolon immediately after the header of a while or for the statement. In a counter-controlled loop, make sure the control variable is incremented (or decremented) in the loop. In a sentinel- controlled loop, make sure the sentinel value is eventually input.
The example uses a do…while statement to print the numbers from 1 to 10. The control variable counter is pre-incremented in the loop-continuation test. Note also the use of the braces to enclose the single-statement body of the do…while.
/*using the do/while repetition statement */
#include <stdio.h>
int main( void )
{
int counter = 1;
do{
printf(“%d”, counter);
} while(++ counter<=10 );
return 0;
}
Output
1 2 3 4 5 6 7 8 9 10
Figure
The figure shows the do…while statement flowchart, which makes it clear that the loop-continuation condition does not execute until after the action is performed at least once.
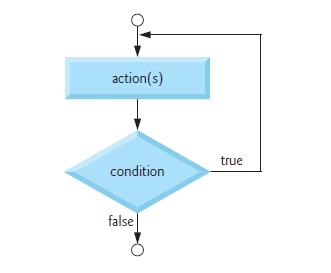
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
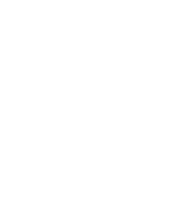