- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
Remaining Functions
The two remaining functions of the string-handling library are strerror and strlen.
Following Table summarizes the strerror and strlen functions.
Function prototype |
Function description |
---|---|
char *strerror( int errornum ); |
Maps errornum into a full-text string in a compiler- and locale-specific manner (e.g. the message may appear in different languages based on its location). A pointer to the string is returned. |
size_t strlen( const char *s ); |
Determines the length of string s. The number of characters preceding the terminating null character is returned. |
Function strerror
Function strerror takes an error number and creates an error message string. A pointer to the string is returned. The example demonstrates strerror.
Example:
/*how to use strerror*/
#include <stdio.h>
#include <string.h>
int main( void )
{
printf( "%s\n", strerror( 2 ) );
return 0;
}
Output:
No such file or directory
Function strlen
Function strlen takes a string as an argument and returns the number of characters in the string the terminating null character is not included in the length. The example demonstrates function strlen.
Example:
/*how to use strerror*/
#include <stdio.h>
#include <string.h>
int main( void )
{
/* initialize 3 char pointers */
const char *string1 = "abcdefghijklmnopqrstuvwxyz";
const char *string2 = "Ricky";
const char *string3 = "Martin";
printf("%s\"%s\"%s%lu\n%s\"%s\"%s%lu\n%s\"%s\"%s%lu\n",
"The length of ", string1, " is ",
( unsigned long ) strlen( string1 ),
"The length of ", string2, " is ",
( unsigned long ) strlen( string2 ),
"The length of ", string3, " is ",
( unsigned long ) strlen( string3 ),
return 0;
}
Output:
The length of "abcdefghijklmnopqrstuvwxyz" is 26
The length of "four" is 5
The length of "Boston" is 6
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
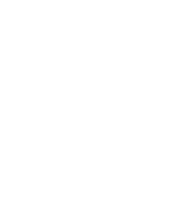