- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
Relational Operators
Relational Operator is used to comparing two quantities and take a certain decision depending on their relation. If the specified relation is true it returns one. If the specified relation is false it returns zero.
Operator |
Description |
Example |
---|---|---|
== |
Checks if the values of two operands are equal or not. If yes, then the condition becomes true. |
(num1 == num2) is not true. |
!= |
Checks if the values of two operands are equal or not. If the values are not equal, then the condition becomes true. |
(num1 != num2) is true. |
> |
Checks if the value of left operand is greater than the value of the right operand. If yes, then the condition becomes true. |
(num1 > num2) is not true. |
< |
Checks if the value of left operand is less than the value of the right operand. If yes, then the condition becomes true. |
(num1 < num2) is true. |
>= |
Checks if the value of left operand is greater than or equal to the value of the right operand. If yes, then the condition becomes true. |
(num1 >= num2) is not true. |
<= |
Checks if the value of left operand is less than or equal to the value of the right operand. If yes, then the condition becomes true. |
(num1 <= num2) is true. |
Example
/* C program for relational operators */
#include <stdio.h>
main()
{
int num1 = 25;
int num2 = 15;
int num3 ;
if( num1 == num2 )
{
printf("Line 1 – num1 is equal to num2\n" );
}
else
{
printf("Line 1 – num1 is not equal to num2\n" );
}
if ( num1 < num2 )
{
printf("Line 2 – num1 is less than num2\n" );
}
else
{
printf("Line 2 – num1 is not less than num2\n" );
}
if ( num1 > num2 )
{
printf("Line 3 – num1 is greater than num2\n" );
}
else
{
printf("Line 3 – num1 is not greater than num2\n" );
}
/* Try with the different values of num1 and num2 */
num1 =15;
num2 = 30;
if ( num1 <= num2 )
{
printf("Line 4 – num1 is either less than or equal to num2\n" );
}
if ( num2 >= num1 )
{
printf("Line 5 – num2 is either greater than or equal to num1\n" );
}
}
Output :
Line 1 – num1 is not equal to num2
Line 2 – num1 is not less than num2
Line 3 – num1 is greater than num2
Line 4 – num1 is either less than or equal to num2
Line 5 – num1 is either greater than or equal to num2
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
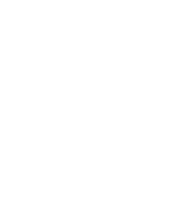