- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
Pointers Operators
The &, or address operator, is a unary operator that returns the address of its operand. For example, assuming the definitions
int y = 5;
int *yPtr;
the statement
yPtr = &y;
assigns the address of the variable y to pointer variable yPtr. Variable yPtr is then said to “point to” y. Figure shows a schematic representation of memory after the preceding assignment is executed.
Figure
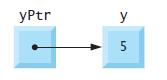
The figure shows the representation of the pointer in memory, assuming that integer variable y is stored at location 600000, and pointer variable yPtr is stored at location 500000. The operand of the address operator must be a variable; the address operator cannot be applied to constants, to expressions or to variables declared with the storage class register.
The unary * operator, commonly referred to as the indirection operator or dereferencing operator, returns the value of the object to which its operand (i.e., a pointer) points. For example, the statement
printf( "%d", *yPtr ); prints the value of variable y, namely 5 in figure. Using * in this manner is called dereferencing a pointer.
Dereferencing a pointer that has not been properly initialized or that has not been assigned to point to a specific location in memory is an error. This could cause a fatal execution time error, or it could accidentally modify important data and allow the program to run to completion with incorrect results.
Example
/*Using the & and * operators */
#include <stdio.h>
.
int main( void )
{
int a; /* a is an integer */
int *aPtr; /* aPtr is a pointer to an integer */
a = 7;
aPtr = &a; /* aPtr set to address of a */
printf( "The address of a is %p"
"\nThe value of aPtr is %p", &a, aPtr );
printf( "\n\nThe value of a is %d"
"\nThe value of *aPtr is %d", a, *aPtr );
printf( "\n\nShowing that * and & are complements of "
"each other\n&*aPtr = %p"
"\n*&aPtr = %p\n", &*aPtr *&aPtr );
return 0;
}
Output :
The address of a is 0012FF7C
The value of aPtr is 0012FF7C
The value of a is 7
The value of *aPtr is 7
Showing that * and & are complements of each other.
&*aPtr = 0012FF7C
*&aPtr = 0012FF7C
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
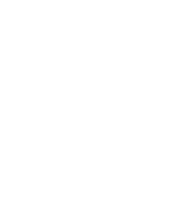