Pointer Expression and Arithmetic
Pointers are valid operands in arithmetic expressions, assignment expressions and comparison expressions. However, not all the operators normally used in these expressions are valid in conjunction with pointer variables. This section describes the operators that can have pointers as operands, and how these operators are used. A limited set of arithmetic operations may be performed on pointers.
A pointer may be incremented (++) or decremented (--), an integer may be added to a pointer (+ or +=),an integer may be subtracted from a pointer (- or -=) and one pointer may be subtracted from another. Assume that array int v[5] has been defined and its first element is at location 3000 in memory. Assume pointer vPtr has been initialized to point to v[0] i.e., the value of vPtr is 3000. illustrates this situation for a machine with 4-byte integers. Variable vPtr can be initialized to point to array v with either of the statements
vPtr = v;
vPtr = &v[ 0 ];
Figure
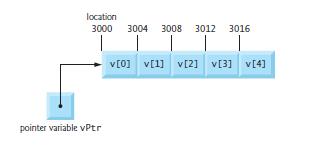
In conventional arithmetic, 3000 + 2 yields the value 3002. This is normally not the case with pointer arithmetic. When an integer is added to or subtracted from a pointer, the pointer is not incremented or decremented simply by that integer, but by that integer times the size of the object to which the pointer refers. The number of bytes depends on the object’s data type.
For example, the statement
vPtr += 2;
would produce 3008 (3000 + 2 * 4), assuming an integer is stored in 4 bytes of memory. In the array v, vPtr would now point to v[2]. If an integer is stored in 2 bytes of memory, then the preceding calculation would result in memory location 3004 (3000 +2 * 2). If the array were of a different data type, the preceding statement would increment the pointer by twice the number of bytes that it takes to store an object of that data type.
When performing pointer arithmetic on a character array, the results will be consistent with regular arithmetic, because each character is 1 byte long.
Figure
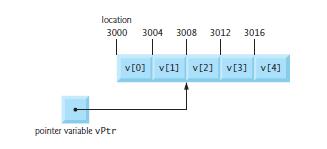
If vPtr had been incremented to 3016, which points to v[4], the state
vPtr -= 4;
would set vPtr back to 3000—the beginning of the array. If a pointer is being incremented or decremented by one, the increment (++) and decrement (--) operators can be used. Either of the statements
++vPtr;
vPtr++;
increments the pointer to point to the next location in the array. Either of the statements
--vPtr;
vPtr--;
decrements the pointer to point to the previous element of the array.
Pointer variables may be subtracted from one another. For example, if vPtr contains the location 3000, and v2Ptr contains the address 3008, the state
x = v2Ptr - vPtr;
would assign to x the number of array elements from vPtr to v2Ptr, in this case, 2 (not 8). Pointer arithmetic is meaningless unless performed on an array. We cannot assume that two variables of the same type are stored contiguously in memory unless they’re adjacent elements of an array.
A pointer can be assigned to another pointer if both have the same type.
The exception to this rule is the pointer to void (i.e., void *), which is a generic pointer that can represent any pointer type. All pointer types can be assigned a pointer to void, and a pointer to void can be assigned a pointer of any type. In both cases, a cast operation is not required.