- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
Nested if…else statements
Nested if…else statements test for multiple cases by placing if…else statements inside if…else statements. For example, the following pseudocode statement will print A for exam grades greater than or equal to 90, B for grades greater than or equal to 80, C for grades greater than or equal to 70, D for grades greater than or equal to 60, and F for all other grades.
if ( grade >= 90 )
printf( "A\n" );
else
if ( grade >= 80 )
printf("B\n");
else
if ( grade >= 70 )
printf("C\n");
else
if ( grade >= 60 )
printf( "D\n" );
else
printf( "F\n" );
If the variable grade is greater than or equal to 90, the first four conditions will be true, but only the printf statement after the first test will be executed. After that printf is executed, the else part of the “outer” if…else statement is skipped. Many C programmers prefer to write the preceding if statement as
if ( grade >= 90 )
printf( "A\n" );
else if ( grade >= 80 )
printf( "B\n" );
else if ( grade >= 70 )
printf( "C\n" );
else if ( grade >= 60 )
printf( "D\n" );
else
printf( "F\n" );
As far as the C compiler is concerned, both forms are equivalent. The latter form is popular because it avoids the deep indentation of the code to the right.
statements contained within a pair of braces is called a compound statement or a block. A compound statement can be placed anywhere in a program that a single statement can be placed. The following example includes a compound statement in the else part of an if…else statement.
if ( grade >= 60 ) {
printf( "Passed.\n" );
} /* end if */
else {
printf( "Failed.\n" );
printf( "You must take this course again.\n" );
} /* end else */
In this case, if a grade is less than 60, the program executes both printf statements in the body of the else and prints Failed. You must take this course again. Notice the braces surrounding the two statements in the else clause. These braces are important. Without the braces, the statement
printf( "You must take this course again.\n" );
would be outside the body of the else part of the if, and would execute regardless of whether the grade was less than 60.Forgetting one or both of the braces that delimit a compound statement.
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
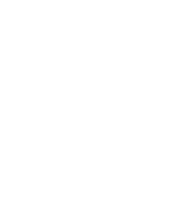