- Introduction to C Programming
- Features And Importance
- Standard Library
- Programming in C
- Data Types
- Variables
- Constants
- Storage Classes
- Static Storage Class
- Scope Rules
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
- Operator Precedence in C
- Control Structures
- Program Controls
- Loop Control Statement
- Functions
- Arrays
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Array Definition
- Array initialization
- Static and Automatic Arrays
- Single Dimensional Array
- Multi Dimensional Array
- Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Definitions and Initialization
- Pointers Operators
- Pointer Expression and Arithmetic
- Pointer-Array Relationship
- Array of Pointers
- Characters and Strings
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Character Handling Library
- String-Conversion Functions
- Standard I/O Functions
- String-Manipulation Functions
- Comparison Functions
- Search Functions
- Memory Functions
- Remaining Functions
- Structures
- typedef
- Unions
- Enumeration Constants
- File Processing
- Preprocessors
- Header Files
- Recursion
- Variable Argument
- Command Line Arguments
- Memory Management
- Typecasting
Logical Operators
These operators are used to perform logical operations on the given expressions. When we use if, for, while then use one condition at once time what happens if we examine condition more than one? In this case, we use logical operators. So logical operators are used to combine two or more condition. There are 3 logical operators in C language. They are, logical AND (&&), logical OR (||) and logical NOT (!).
Operator |
Description |
Example |
---|---|---|
&& |
Logical AND operator. If both the operands are non-zero, then the condition becomes true. |
(num1 && num2) is false. |
|| |
Logical OR Operator. If any of the two operands is non-zero, then the condition becomes true |
(num1 || num2) is true. |
! |
Logical NOT Operator. It is used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make it false. |
!(num1 && num2) is true. |
Example
/* A C program for logical operator */
#include <stdio.h>
main()
{
int num1 = 5;
int num2 = 20;
int num3 ;
if ( num1 && num2 )
{
printf("Line 1 - Condition is true\n" );
}
if ( num1 || num2 )
{
printf("Line 2 - Condition is true\n" );
}
/* lets change the value of a and b */
num1 = 0;
num2 = 10;
if ( num1 && num2 )
{
printf("Line 3 - Condition is true\n" );
}
else
{
printf("Line 3 - Condition is not true\n" );
}
if ( !(num1 && num2) )
{
printf("Line 4 - Condition is true\n" );
}
}
Output:
Line 1 - Condition is true
Line 2 - Condition is true
Line 3 - Condition is not true
Line 4 - Condition is true
JOIN TUTORIALS LINK
Our Newsletter Will Let You Know When Any New
Articles, Tutorials and Video Are Released.
CONTRIBUTE
MOBILE APP
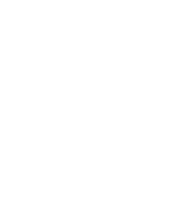